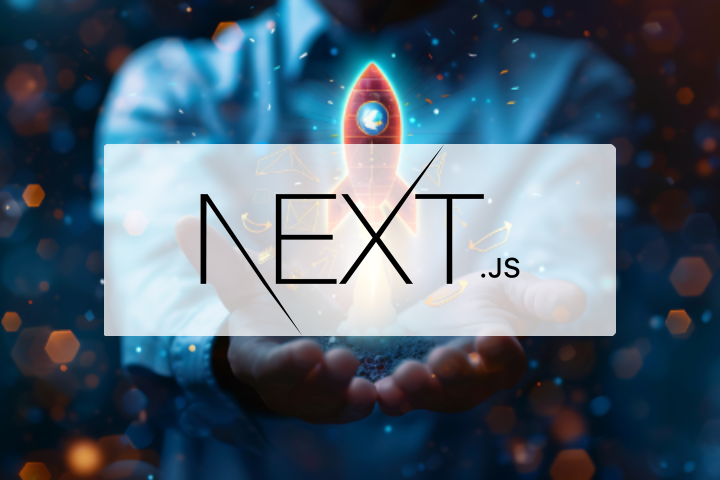
When running a Next.js application with PM2 on an Ubuntu server, setting the environment variable directly in the command line might not always work as expected due to the way PM2 manages processes. Here’s how you can make sure your application starts on a specific port:
Use PM2 Environment Configuration
The most reliable way to set environment-specific configurations with PM2 is to use an ecosystem configuration file. This method allows you to define various environment variables and more using a structured JSON or JavaScript format.
Step 1: Create an Ecosystem File
-
Create a file named
ecosystem.config.js
(or use a similar name) in your project directory. -
Define your application and specify the environment variables like
PORT
:module.exports = { apps: [ { name: 'your-app-name', script: 'npm', args: 'run start', env: { PORT: 4000 } } ] };
Step 2: Start Your Application with PM2
Use the ecosystem file to start your application:
pm2 start ecosystem.config.js
This command will read the configuration from the file and start your Next.js application with the specified environment variables.
Alternative: Use a .env
File and Load It
If you prefer to stick with using .env
files or use a different way to manage configuration, ensure your .env
file is being read properly in the context of the application start command. While not managed directly by PM2, here is a common approach:
-
Install dotenv package:
You need to make sure your app can load environment variables from a
.env
file. Use thedotenv
package if necessary:npm install dotenv
-
Load
.env
in Next.js scripts:Modify your
package.json
or wherever your Node.js startup logic is defined to load the.env
configuration:For an example using a script:
"scripts": { "start": "node -r dotenv/config server.js" }
Then, start your application with PM2:
pm2 start npm --name 'your-app-name' -- run start
Double-check that:
- Your
ecosystem.config.js
(or.env
management) is correctly placed in the directory where you run the PM2 command. - Your server listens on the specified port.
- There are no conflicts with other processes using the same port.
Using the ecosystem configuration is generally the most straightforward and maintainable approach when working with PM2 on a server such as Ubuntu.