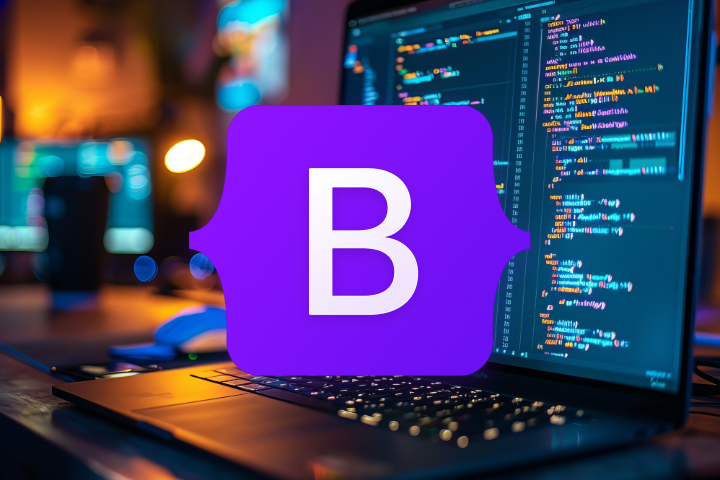
Here is a WordPress query to get all children of a specific page by its ID, and then loop through those children to dynamically populate the Bootstrap carousel items in the HTML:
- First, you need to get the child pages of a specific parent page ID.
- Loop through those child pages and dynamically generate the carousel items.
Here’s how you can do it:
<?php
$page_id = 42; // Replace with your parent page ID
// Get the child pages
$args = array(
'post_type' => 'page',
'posts_per_page' => -1,
'post_parent' => $page_id,
'orderby' => 'menu_order',
'order' => 'ASC',
);
$child_pages = new WP_Query($args);
?>
<div class="container-fluid">
<div class="row g-0">
<div class="col-12">
<div id="carouselExampleControls" class="carousel slide" data-bs-ride="carousel">
<div class="carousel-inner">
<?php if ($child_pages->have_posts()): ?>
<?php $item_count = 0; ?>
<?php while ($child_pages->have_posts()): $child_pages->the_post(); ?>
<div class="carousel-item text-center <?php echo $item_count === 0 ? 'active' : ''; ?> item-<?php echo $item_count + 1; ?>">
<div class="content">
<h1><?php the_title(); ?></h1>
<p><?php the_excerpt(); ?></p>
<button class="btn btn-light text-dark" onclick="window.location.href='<?php the_permalink(); ?>'">Learn More</button>
</div>
</div>
<?php $item_count++; ?>
<?php endwhile; ?>
<?php else: ?>
<p>No child pages found.</p>
<?php endif; ?>
</div>
</div>
</div>
</div>
</div>
<?php wp_reset_postdata(); ?>
Explanation:
$page_id
: Replace this with the ID of your parent page.$args
: This array sets up the query to retrieve child pages of the specified parent page, ordered by menu order in ascending order.$child_pages
: This is the WP_Query object that holds the query results.- The
while
loop: Iterates through the child pages, outputting the carousel items. item_count
: This variable tracks the index of the carousel items to set the first one asactive
.
This code assumes you have child pages under a parent page and that you want to use the title and excerpt of each child page in the carousel. Adjust the HTML and PHP as necessary to suit your specific requirements.