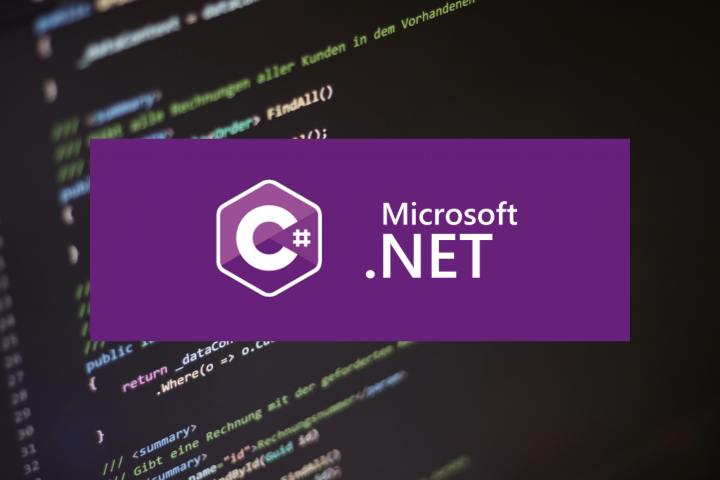
To manage .NET 8.0 applications on an Ubuntu server, you can use systemd
to create a service for your application, which allows you to start, stop, and manage the lifecycle of your application in a way similar to how pm2
works for Node.js applications.
Here are the steps to accomplish this:
Prerequisites
-
Install .NET SDK/Runtime: Ensure that the appropriate .NET runtime is installed on your server. You can install it by following our post on How to Install .NET 8 on Ubuntu.
-
Publish Your Application: Before deploying your application, publish it. This can be done using:
dotnet publish -c Release -o /path/to/output
Replace
/path/to/output
with your desired output directory. -
Make Your Application Executable: If your app is a console app, you typically run it using
dotnet YourApp.dll
. Ensure the application is executable if needed:chmod +x /path/to/output/YourApp
Using systemd
to Manage Your Application
-
Create a Systemd Service File
Create a new service file for your application. You could name it something like
myapp.service
. Open a text editor and create it in the/etc/systemd/system/
directory:sudo nano /etc/systemd/system/myapp.service
-
Define the Service Configuration
Add the following configuration to your service file:
[Unit] Description=My .NET Application After=network.target [Service] WorkingDirectory=/path/to/output ExecStart=/usr/bin/dotnet /path/to/output/YourApp.dll Restart=always RestartSec=10 KillSignal=SIGINT SyslogIdentifier=myapp User=www-data Environment=ASPNETCORE_ENVIRONMENT=Production Environment=DOTNET_PRINT_TELEMETRY_MESSAGE=false [Install] WantedBy=multi-user.target
Adjust the
WorkingDirectory
,ExecStart
, andUser
settings as needed based on your environment and the location of your application. -
Reload systemd and Start the Service
Reload
systemd
to make it aware of the new service:sudo systemctl daemon-reload
Start your service and enable it to start on boot:
sudo systemctl start myapp.service sudo systemctl enable myapp.service
-
Managing the Service
You can now manage your service using the standard
systemd
commands:-
Start the application:
sudo systemctl start myapp.service
-
Stop the application:
sudo systemctl stop myapp.service
-
Restart the application:
sudo systemctl restart myapp.service
-
Check the status of the application:
sudo systemctl status myapp.service
-
Logging
The logs for the service will typically be available through journalctl
. You can view them using:
sudo journalctl -u myapp.service -f
This will show the log for your specific service in real-time. Adjust settings in your service file if you need more customized logging.
By setting up your .NET application as a systemd
service, you have a robust mechanism for process management on your Ubuntu server that provides similar capabilities to pm2
for Node.js applications.