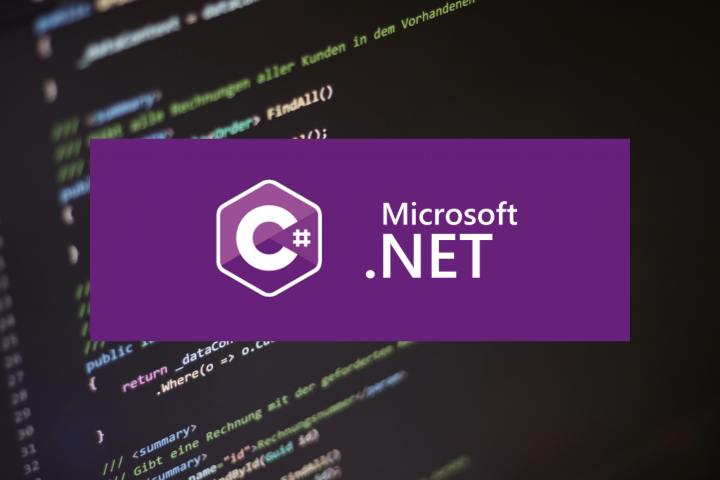
To set up a .NET 8 API to run on an Ubuntu Apache server, you’ll need to configure the server as a reverse proxy to manage your .NET application. Below are the steps to guide you through the process:
Prerequisites
-
Install .NET 8 SDK
Ensure you have the .NET 8 SDK installed on your Ubuntu server.wget https://dot.net/v1/dotnet-install.sh -O dotnet-install.sh bash dotnet-install.sh --channel 8.0
Or follow the our post on How to Install .Net 8 on Ubuntu.
-
Install Apache
Ensure Apache is installed and running.sudo apt update sudo apt install apache2
-
Install required Apache modules
Ensure that mod_proxy, mod_proxy_http, and mod_ssl (if needed) are enabled.sudo a2enmod proxy sudo a2enmod proxy_http sudo a2enmod ssl # If you plan on using HTTPS sudo systemctl restart apache2
Publish Your .NET 8 API
-
Publish your .NET API
Navigate to your project folder and publish it:
dotnet publish --configuration Release
Your publish output will be in the
bin/Release/net8.0/publish
folder.
Run Your .NET Application
-
Navigate to the publish folder and run your application
cd bin/Release/net8.0/publish dotnet YourApp.dll
Confirm that your application is running on the intended port, usually the default port 5000.
Configure Apache
-
Create an Apache configuration file for your site
Create a new configuration file in
/etc/apache2/sites-available/
. For example:sudo nano /etc/apache2/sites-available/yourapp.conf
Insert the following configuration, modifying it to suit your server environment:
<VirtualHost *:80> ServerName yourdomain.com ProxyPreserveHost On ProxyPass / http://localhost:5000/ ProxyPassReverse / http://localhost:5000/ ErrorLog ${APACHE_LOG_DIR}/yourapp-error.log CustomLog ${APACHE_LOG_DIR}/yourapp-access.log combined </VirtualHost>
If you plan to use HTTPS, consider using
mod_ssl
and configure a corresponding virtual host for port 443. You’ll need a valid SSL certificate, which can be obtained for free from Let’s Encrypt:<VirtualHost *:443> ServerName yourdomain.com ProxyPreserveHost On ProxyPass / http://localhost:5000/ ProxyPassReverse / http://localhost:5000/ ErrorLog ${APACHE_LOG_DIR}/yourapp-error.log CustomLog ${APACHE_LOG_DIR}/yourapp-access.log combined SSLEngine on SSLCertificateFile /path/to/certificate.crt SSLCertificateKeyFile /path/to/privatekey.key SSLCertificateChainFile /path/to/chainfile.pem </VirtualHost>
-
Enable the new site configuration
Enable the new configuration and disable the default site if needed:
sudo a2ensite yourapp.conf sudo a2dissite 000-default.conf sudo systemctl reload apache2
Test Your Setup
-
Access your application
Open a web browser and navigate to
http://yourdomain.com
(orhttps://yourdomain.com
if using SSL). Ensure your application loads as expected. -
Troubleshoot if necessary
Check Apache logs:
sudo tail -f /var/log/apache2/error.log sudo tail -f /var/log/apache2/access.log
This setup should enable your .NET 8 API to run behind an Apache server on Ubuntu. Be sure to replace placeholders like yourdomain.com
and paths with your actual domain and certificate paths.