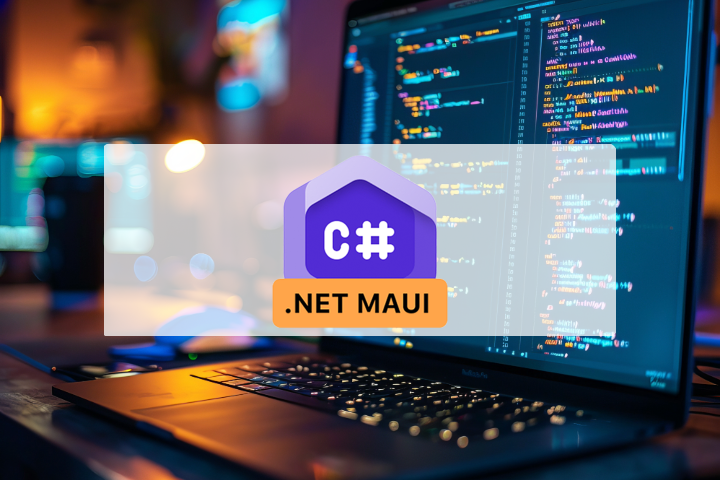
Hello all! Today, I want to share a simple yet effective way to manage app store package IDs in your C# .Net Maui Application. Whether you’re an indie developer or part of a larger team, keeping track of your app’s unique identifiers across various platforms can be quite the challenge. We often need a structured way to store and retrieve these IDs with ease. Let me show you how I’ve tackled this in my projects and why this approach could be beneficial for your own.
The Problem
Every app deployment across different app stores requires unique identifiers. When your application is available in the Microsoft Store, Apple App Store, and Google Play Store, each platform assigns its own ID to your app. These IDs are essential for a myriad of functions such as in-app purchases, updates, and analytics integration.
Managing these identifiers can become cumbersome, especially if they’re not well-organized. The traditional approach of hardcoding IDs throughout your application not only invites errors but also makes maintenance a nightmare. So, how do we manage these IDs effectively?
The Solution
The idea is simple: use a class to encapsulate these key-value pairs for each of your package IDs. By doing so, you create a single source of truth for your app store identifiers.
Here’s a quick recap of the class structure we define:
public class PackageIdMapping
{
public string PackageId { get; set; }
public string MicrosoftStoreId { get; set; }
public string AppleStoreId { get; set; }
public string GooglePlayStoreId { get; set; }
public PackageIdMapping(string packageId, string microsoftStoreId, string appleStoreId, string googlePlayStoreId)
{
PackageId = packageId;
MicrosoftStoreId = microsoftStoreId;
AppleStoreId = appleStoreId;
GooglePlayStoreId = googlePlayStoreId;
}
}
Why This Approach?
-
Clarity and Maintenance: By encapsulating the data, you have a clear and concise representation of all your app’s identifiers. Adding or updating store IDs becomes just a matter of tweaking this class.
-
Scalability: As your app expands into additional markets or stores, you just extend this setup. No more digging through multiple sections of your codebase to align identifiers.
-
Flexibility: Storing these mappings in a List allows you to easily perform operations such as searching, adding, removing, or updating mappings.
-
Centralized Management: All your identifiers are in one place, which simplifies both documentation and debugging.
Real-Life Example
Let’s see how we can put this into action. Suppose you have several different versions or builds of an app:
var mappings = new List<PackageIdMapping>
{
new PackageIdMapping("package-1", "microsoft-1", "apple-1", "google-1"),
new PackageIdMapping("package-2", "microsoft-2", "apple-2", "google-2"),
new PackageIdMapping("package-3", "microsoft-3", "apple-3", "google-3")
};
// Retrieve details for a specific package
var mapping = mappings.FirstOrDefault(m => m.PackageId == "package-1");
if (mapping != null)
{
Console.WriteLine($"Microsoft Store ID: {mapping.MicrosoftStoreId}");
Console.WriteLine($"Apple Store ID: {mapping.AppleStoreId}");
Console.WriteLine($"Google Play Store ID: {mapping.GooglePlayStoreId}");
}
else
{
Console.WriteLine("Package ID not found.");
}
Conclusion
Overall, using a class to manage your app store IDs ensures your code remains clean and manageable. It’s a practical approach that I’ve found invaluable. If you’re dealing with multiple apps or platforms, this method can save you loads of time and reduce potential errors—from my keyboard to yours.
Thanks for reading, and until next time, keep coding!