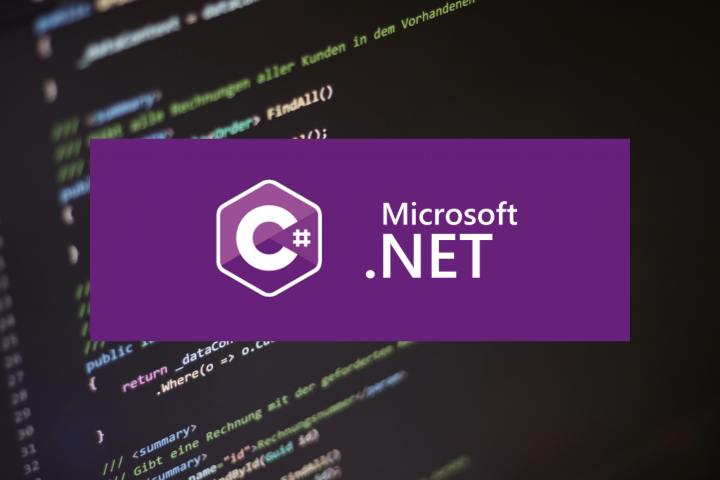
To implement speech-to-text functionality in a .NET MAUI Blazor Hybrid application, you can leverage platform-specific speech recognition APIs or third-party services. Here’s how you might approach this task:
Using Platform-Specific APIs
For a .NET MAUI Blazor Hybrid app, you can use platform-specific features by creating platform-specific implementations. Here’s a brief overview of how you might implement speech-to-text functionality on different platforms:
iOS
- Use the
AVSpeechSynthesizer
andSFSpeechRecognizer
classes from theAVFoundation
framework.
Android
- Use
SpeechRecognizer
API to capture and convert speech to text.
Windows
- Access Windows’s built-in speech recognition capabilities like using
Windows.Media.SpeechRecognition
.
Implementing Speech-to-Text
-
Create Platform-Specific Services
Define an interface for your service:
public interface ISpeechToTextService { Task<string> RecognizeSpeechAsync(); }
-
Implement on Each Platform
For example, on Android:
using Android.Speech; using Microsoft.Maui.Controls; using MyApp.Platforms.Android; using MyApp.Services; using System.Threading.Tasks; [assembly: Dependency(typeof(SpeechToTextService))] namespace MyApp.Platforms.Android { public class SpeechToTextService : ISpeechToTextService { public Task<string> RecognizeSpeechAsync() { // Implementation using Android's SpeechRecognizer here } } }
Similarly, create implementations for iOS and Windows using their respective APIs.
-
Consume the Service in Your Blazor Component
In your Blazor component, use the defined service:
@inject ISpeechToTextService SpeechToTextService <button @onclick="StartSpeechRecognition">Start Speech Recognition</button> <p>Recognized Text: @recognizedText</p> @code { private string recognizedText; private async Task StartSpeechRecognition() { try { recognizedText = await SpeechToTextService.RecognizeSpeechAsync(); } catch (Exception ex) { // Handle exceptions } } }
Using Third-Party Speech-to-Text Services
If platform-specific APIs are too complex or not sufficient, you can use cloud services like:
- Google Cloud Speech-to-Text API
- Azure Speech Services
- IBM Watson
These services typically require sending audio streams to their servers and interpreting the results.
- Record Audio: Capture audio input on the device.
- Send to API: Use an HTTP client to send the audio data to the chosen service.
- Receive Text: Receive and process the text response from the service.
Conclusion
Implementing speech-to-text in a .NET MAUI Blazor Hybrid application involves leveraging platform-specific APIs or third-party cloud services. Platform-specific services require some P/Invoke or Dependency Injection, while cloud services may require HTTP requests to their endpoints. This approach allows you to have greater control tailored to your application’s requirements.